In my exploration of WebGL, I encountered a common issue: running out of memory.
Asset Data Management
1. Optimize your assets: Reduce the size of your assets by compressing textures, simplifying 3D models, or using lower-resolution images. This helps conserve memory and improves performance.
2. Unload unused assets: If your WebGL application uses a large number of assets, make sure to unload assets that are no longer needed. This frees up memory for other assets to be loaded.
3. Implement a caching strategy: Caching frequently used assets can significantly improve performance. Consider using a virtual file system or caching system to efficiently manage asset loading and unloading.
4. Monitor memory usage: Keep an eye on your application’s memory usage during runtime. WebGL provides APIs to check the available memory and can help you identify potential memory leaks or excessive memory usage.
5. Consider using a memory management library: Libraries like Unity or other memory management frameworks can assist with efficient asset data management in WebGL applications.
Reducing Memory Usage
To reduce memory usage in WebGL, follow these tips:
1. Optimize your code: Review your source code and identify areas where you can optimize memory usage. Look for unnecessary variables or data structures that can be removed or simplified.
2. Use efficient data structures: Choose data structures that are optimized for memory usage. For example, use arrays instead of dictionaries or maps when possible.
3. Minimize texture and mesh size: Use smaller textures and meshes to reduce memory usage. Consider using compression techniques to further reduce the size of your assets.
4. Unload unused assets: Be mindful of the assets you are loading into memory. Unload any assets that are no longer needed to free up memory.
5. Implement memory management: Use techniques like object pooling or resource recycling to efficiently manage memory usage. Reuse objects instead of creating new ones whenever possible.
6. Limit the number of draw calls: Reducing the number of draw calls can help optimize memory usage. Combine multiple objects into a single mesh whenever feasible.
7. Consider using a lower resolution: If your application allows for it, consider using a lower resolution for textures and renderings. This can significantly reduce memory usage.
8. Profile and optimize: Use profiling tools to identify memory bottlenecks and optimize your code accordingly. Unity’s built-in profiler can help you pinpoint areas that consume excessive memory.
Garbage Collection Strategies
1. Object Pooling: Reusing objects instead of creating new ones can significantly reduce memory allocation. Implement object pooling for frequently used objects, such as game entities or particles.
2. Dispose Unused Objects: Explicitly release resources and memory occupied by objects that are no longer needed. Use the “dispose” method to clean up WebGL objects like textures, buffers, or shaders.
3. Minimize Texture Usage: Textures consume a significant amount of memory. Optimize their usage by reusing textures, resizing them to the necessary dimensions, and compressing them when possible.
4. Reduce Memory Leaks: Be mindful of circular references between objects, as they can prevent garbage collection. Use weak references or break the circular references manually to ensure proper memory management.
5. Use Efficient Data Structures: Choose data structures that minimize memory overhead and improve access speed. Arrays are generally more memory-efficient than dictionaries or lists.
6. Monitor Memory Usage: Keep track of memory consumption during runtime to identify potential issues. Use browser tools, such as the Chrome DevTools, to analyze memory usage and detect memory leaks.
7. Optimize JavaScript Code: Write efficient JavaScript code to minimize memory usage. Avoid excessive object creation, use typed arrays for efficient buffer manipulation, and optimize loops and conditionals.
By implementing these garbage collection strategies, you can improve memory management in WebGL applications, reduce the risk of running out of memory, and optimize overall performance. For more detailed information and examples, refer to relevant resources like the Unity documentation or WebGL programming guides.
Memory Constraints and Solutions
Memory Constraint | Solution |
---|---|
Limited GPU Memory | 1. Optimize texture usage by reducing texture size, using texture compression, or employing texture atlases. 2. Use lower precision data types where applicable. 3. Implement dynamic memory management to load/unload resources based on demand. 4. Prioritize and reuse memory for frequently accessed resources. |
Excessive Memory Allocations | 1. Avoid unnecessary memory allocations by reusing existing objects or buffers. 2. Implement object pooling to manage and reuse memory for frequently created objects. 3. Use typed arrays for efficient memory management and data processing. |
Memory Leaks | 1. Properly release resources when they are no longer needed. 2. Use tools like WebGL Inspector or Chrome DevTools to identify and fix memory leaks. 3. Implement reference counting or garbage collection mechanisms to manage memory. |
Large Data Sets | 1. Employ level-of-detail techniques to render only necessary details based on distance or visibility. 2. Use streaming or chunking techniques to load data progressively. 3. Implement data compression algorithms to reduce memory footprint. |
Error Detection and Best Practices
1. Monitor Memory Usage: Keep an eye on your memory usage throughout development and testing. Use tools like Unity’s Profiler or your operating system’s task manager to track memory consumption. This will help you identify potential memory leaks or excessive memory usage.
2. Optimize Textures: Large textures consume a significant amount of memory. Resize and compress textures to reduce their memory footprint. Avoid using unnecessary high-resolution textures or textures larger than necessary for your application.
3. Efficient Resource Management: Properly manage resources like shaders, meshes, and materials. Reuse and release resources when they are no longer needed to free up memory.
4. Limit Draw Calls: Reducing the number of draw calls can improve performance and reduce memory usage. Batch similar objects together to minimize draw calls.
5. Use Object Pooling: Instead of constantly creating and destroying objects, implement object pooling. This technique reuses objects, reducing memory allocation and garbage collection overhead.
6. Minimize JavaScript Overhead: Be mindful of the JavaScript code you run in WebGL. Excessive computations or inefficient coding practices can strain memory resources. Optimize your scripts and use performance-conscious coding techniques to minimize memory usage.
7. Clear Unnecessary Data: When you no longer need certain data or objects, make sure to clear them from memory. This includes textures, buffers, and other resources that are no longer in use.
FAQs
What is out of memory error?
An out of memory error refers to a situation where the Database Node Memory (KB) falls below 2 percent of the target size, causing the inability to free up database pages on the node.
What is the initial memory size in unity WebGL?
The initial memory size in Unity WebGL is determined by TOTAL_MEMORY, which is defined in the Player Settings. The default value is 256mb, but an empty project can work with just 16mb. However, most real-world content will require more memory, such as 256 or 386mb in most cases.
How do I allocate less memory to WebGL?
To allocate less memory to WebGL, you can utilize AssetBundles to pack your asset data. AssetBundles provide the ability to have complete control over your asset downloads, thereby reducing memory usage.
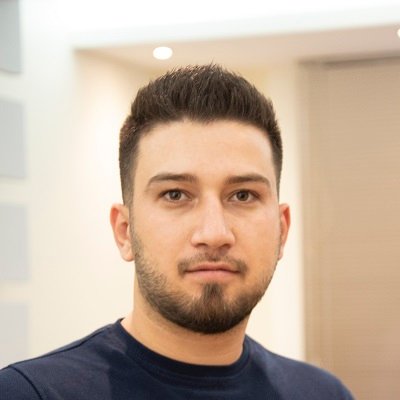