In this article, we delve into the world of stack overflow errors in Java, exploring their causes and providing preventive measures.
Understanding Causes
Understanding the causes of stack overflow errors in Java is crucial for preventing and troubleshooting these issues.
One common cause is excessive recursion, where a function calls itself repeatedly without a base case to stop the recursion. This can quickly fill up the call stack and cause a stack overflow error. To prevent this, make sure your recursive functions have a well-defined termination condition.
Another cause is buffer overflow, which occurs when a program writes data beyond the boundaries of a buffer. This can lead to memory corruption and stack overflow errors. Always ensure that you are using proper bounds checking when working with arrays or buffers in Java.
Integer overflow is another potential cause. When an integer value exceeds its maximum limit, it wraps around to the minimum value, which can lead to unexpected behavior and stack overflow errors. Be mindful of the range of values that your variables can hold and handle potential overflow scenarios appropriately.
Additionally, recursive functions with a high exponential time complexity can also cause stack overflow errors. If your program performs computationally intensive tasks, consider optimizing the code to reduce the time complexity and prevent stack overflow errors.
Identifying Infinite and Deep Recursion
Infinite and Deep Recursion:
Identifying infinite and deep recursion is crucial for understanding and preventing stack overflow errors in Java. Recursion is a programming technique where a function calls itself, and it can lead to stack overflow errors when the call stack exceeds its memory limit.
To identify infinite recursion, look for a condition that should terminate the recursive function but doesn’t. This could be due to a missing base case or incorrect termination condition. Review the recursive function’s logic and ensure that it will eventually reach a base case and terminate.
Deep recursion, on the other hand, occurs when the recursion goes too deep, consuming excessive stack memory. To identify deep recursion, analyze the number of recursive calls made and the amount of stack memory used. If the number of recursive calls or the stack memory usage is significantly high, consider optimizing the recursive function or using an iterative approach instead.
By identifying and addressing infinite and deep recursion, you can prevent stack overflow errors and ensure the smooth execution of your Java programs.
Analyzing Large Stack Variables
Variable Name | Data Type | Size | Description |
---|---|---|---|
array | int[] | 1 MB | An array of integers used for data processing. |
matrix | double[][] | 5 MB | A two-dimensional matrix for complex calculations. |
str | String | 50 KB | A string variable storing user input. |
object | CustomObject | 10 KB | An instance of a custom-defined class. |
buffer | byte[] | 500 KB | A buffer for reading data from a file. |
Exploring Error Handling Methods
- Try-Catch Blocks: Use try-catch blocks to catch and handle exceptions that may occur during the execution of your code.
- Throwing Exceptions: Use the throw keyword to manually throw an exception when a specific error condition is encountered.
- Checked Exceptions: Handle checked exceptions by either catching them or declaring them in your method signature.
- Uncaught Exception Handlers: Implement an uncaught exception handler to handle any exceptions that are not caught within your code.
- Stack Traces: Analyze stack traces to identify the cause of the error and understand the sequence of method calls leading up to the error.
- Logging: Use logging frameworks like Log4j or java.util.logging to log error messages and provide valuable information for debugging.
- Custom Exception Classes: Create custom exception classes to represent specific error conditions in your code and handle them appropriately.
- Exception Chaining: Utilize exception chaining to provide additional context and information about the root cause of an exception.
- Handling Out of Memory Errors: Implement strategies to handle OutOfMemoryError, such as optimizing memory usage or gracefully shutting down the application.
F.A.Qs
What does stack error mean?
A stack error means that the capacity of a stack has been exceeded when attempting a complex calculation.
What does stack overflow do?
Stack Overflow is a website that serves as a platform for computer programmers to ask and answer questions about various programming topics. It was created in 2008 by Jeff Atwood and Joel Spolsky.
How do you prevent stack overflow error?
To prevent stack overflow errors, it is crucial to include a base case in recursive functions. Without a base case, the function calls will continue indefinitely, leading to a stack overflow. Including a base case ensures that the recursive calls eventually stop.
How do you fix a stack overflow error?
To fix a stack overflow error, you should carefully inspect the stack trace to identify the recurring pattern of line numbers. This will help you locate the code causing problematic recursion.
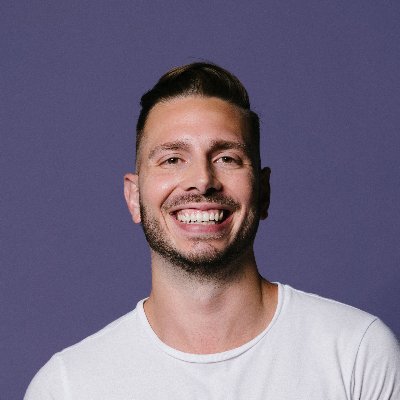